_ _
(_) ___ | |_ ____
| |/ _ \| __|_ /
| | (_) | |_ / /
_/ |\___/ \__/___|
|__/
Julia Set experiments
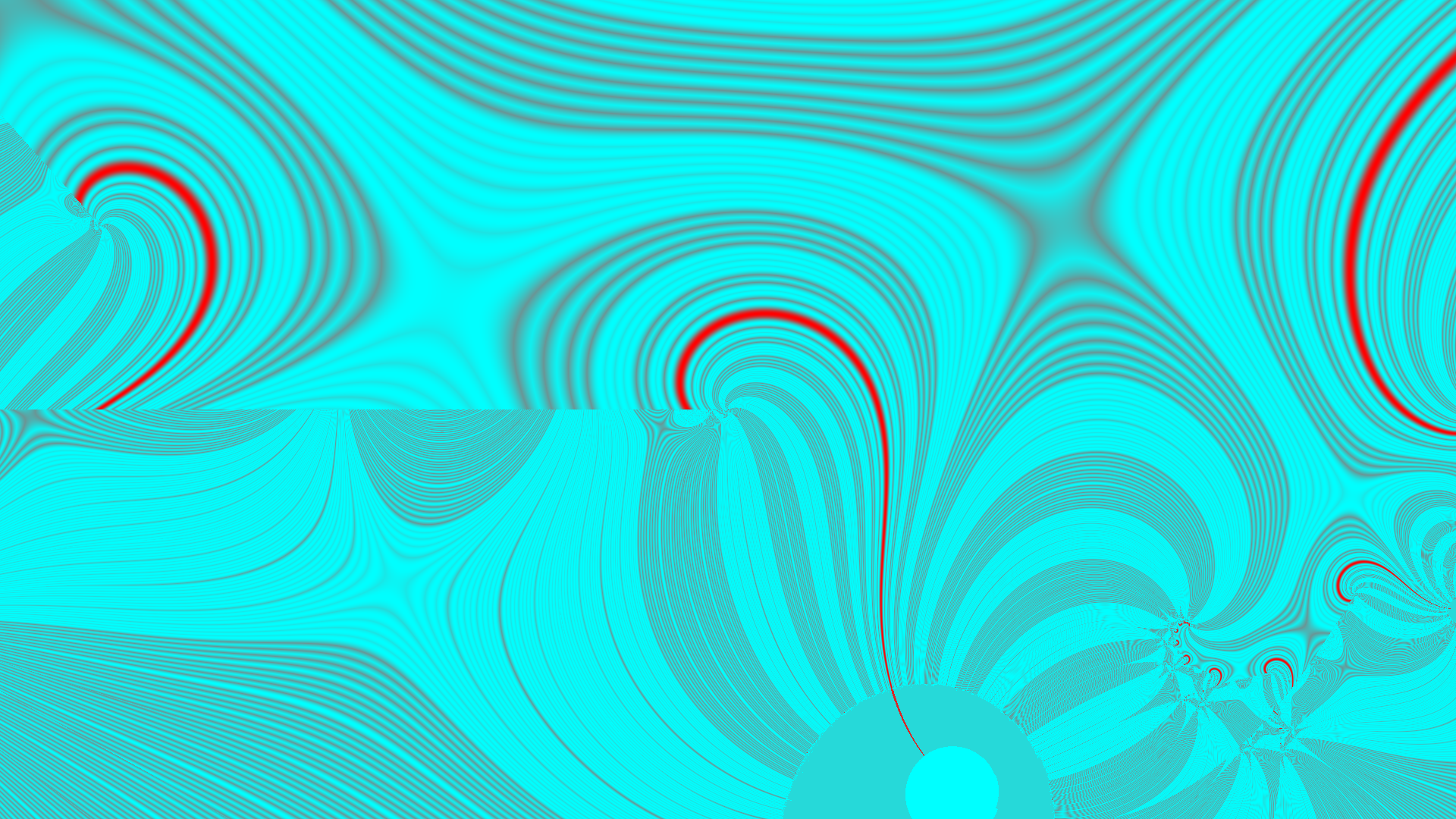
//
// fractal.c - written by Ted Burke - 8-Mar-2023
//
// gcc -o fractal fractal.c -lm
//
#include <stdio.h>
#include <complex.h>
#include <stdlib.h>
#include <stdint.h>
#include <math.h>
#define W 1920
#define H 1080
uint8_t p[H][W];
int main()
{
int x, y, n;
complex double z, c, zz;
uint8_t *p = malloc(W*H*3);
c = -0.625 - 0.4*I;
for (y=0 ; y<H ; ++y)
{
for (x=0 ; x<W ; ++x)
{
z = 0.001 * (x-W/2 + I*(y-H/2));
for (n=0 ; n<16 ; ++n)
{
zz = cpow(z, 0.555555);
z = (z*z + c)/(zz + c);
}
uint8_t pp = 128.0 + 127.0*cos(carg(z));
p[3*(y*W+x)+0] = pp;
p[3*(y*W+x)+2] = 255 - pp;
p[3*(y*W+x)+1] = 255 - pp;
}
}
FILE *f = fopen("fractal.pgm", "wb");
fprintf(f, "P6\n%d %d\n255\n", W, H);
fwrite(p, W*H*3, 1, f);
fclose(f);
free(p);
return 0;
}
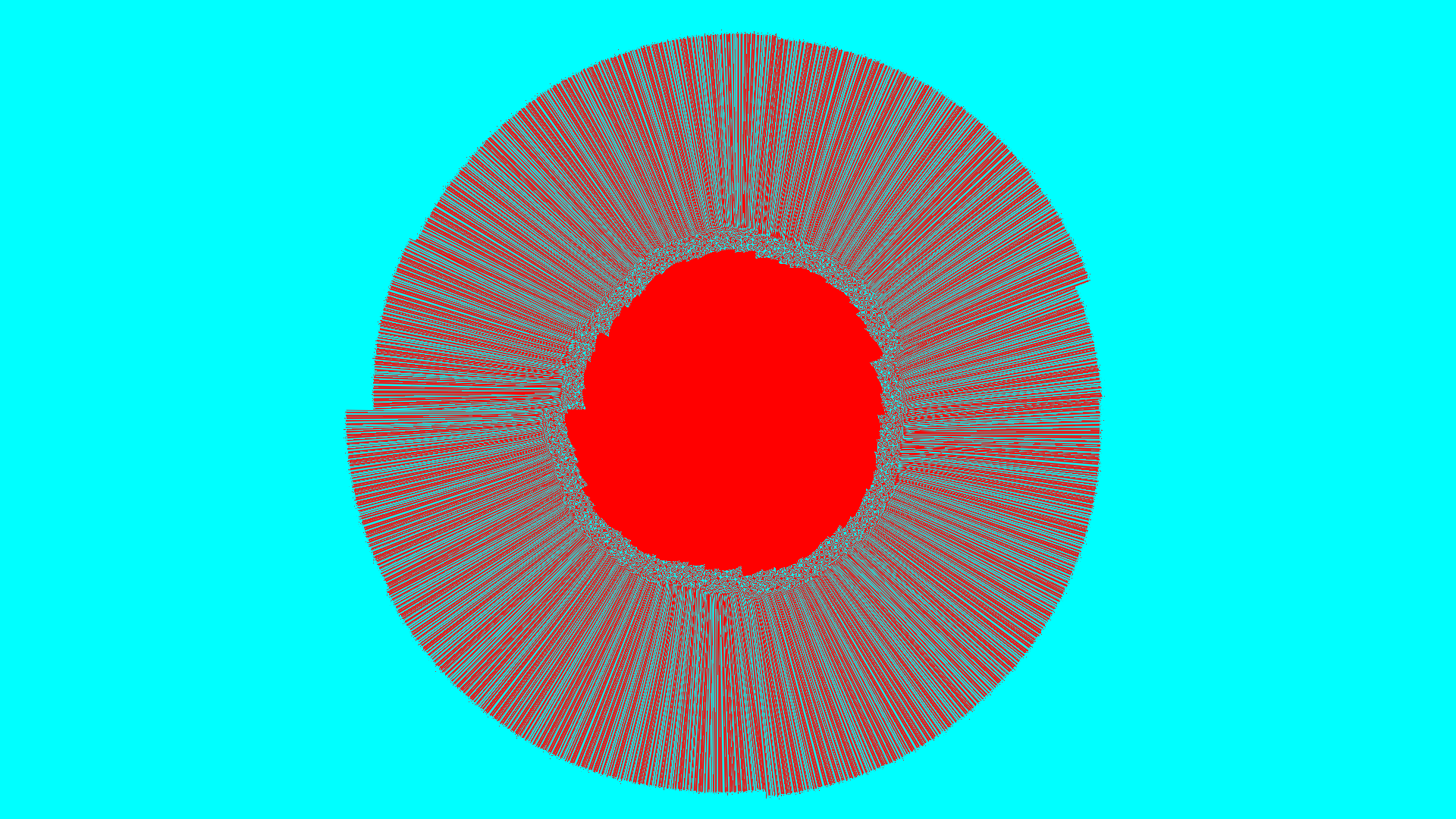
//
// fractal.c - written by Ted Burke - 8-Mar-2023
//
// gcc -o fractal fractal.c -lm
//
#include <stdio.h>
#include <complex.h>
#include <stdlib.h>
#include <stdint.h>
#include <math.h>
#define W 1920
#define H 1080
uint8_t p[H][W];
int main()
{
int x, y, n;
complex double z, c, zz;
uint8_t *p = malloc(W*H*3);
c = -0.625 - 0.4*I;
for (y=0 ; y<H ; ++y)
{
for (x=0 ; x<W ; ++x)
{
z = 0.035 * (x-W/2 + I*(y-H/2));
for (n=0 ; n<16 ; ++n)
{
zz = cpow(z, 0.5);
z = (z*z * c)/(2*zz - c);
}
uint8_t pp = 128.0 + 127.0*cos(carg(z));
p[3*(y*W+x)+0] = pp;
p[3*(y*W+x)+2] = 255 - pp;
p[3*(y*W+x)+1] = 255 - pp;
}
}
FILE *f = fopen("fractal.pgm", "wb");
fprintf(f, "P6\n%d %d\n255\n", W, H);
fwrite(p, W*H*3, 1, f);
fclose(f);
free(p);
return 0;
}
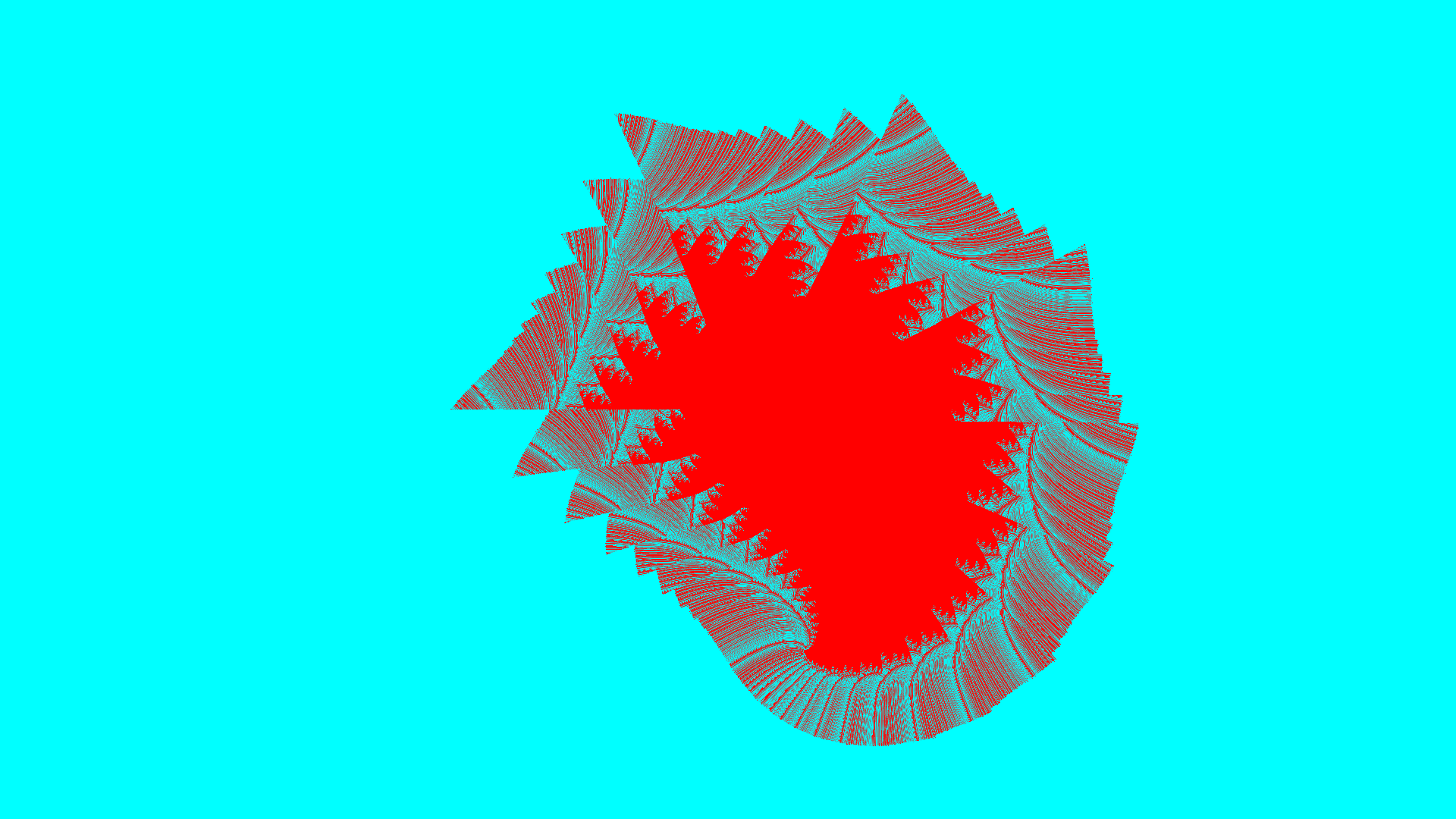
//
// fractal.c - written by Ted Burke - 8-Mar-2023
//
// gcc -o fractal fractal.c -lm
//
#include <stdio.h>
#include <complex.h>
#include <stdlib.h>
#include <stdint.h>
#include <math.h>
#define W 1920
#define H 1080
uint8_t p[H][W];
int main()
{
int x, y, n;
complex double z, c, zz;
uint8_t *p = malloc(W*H*3);
c = 0.625 - 0.4*I;
for (y=0 ; y<H ; ++y)
{
for (x=0 ; x<W ; ++x)
{
z = 0.012 * (x-W/2 + I*(y-H/2));
for (n=0 ; n<32 ; ++n)
{
zz = cpow(z, 0.75);
z = (z*z * c)/(zz + c);
}
uint8_t pp = 128.0 + 127.0*cos(carg(z));
p[3*(y*W+x)+0] = pp;
p[3*(y*W+x)+2] = 255 - pp;
p[3*(y*W+x)+1] = 255 - pp;
}
}
FILE *f = fopen("fractal.pgm", "wb");
fprintf(f, "P6\n%d %d\n255\n", W, H);
fwrite(p, W*H*3, 1, f);
fclose(f);
free(p);
return 0;
}
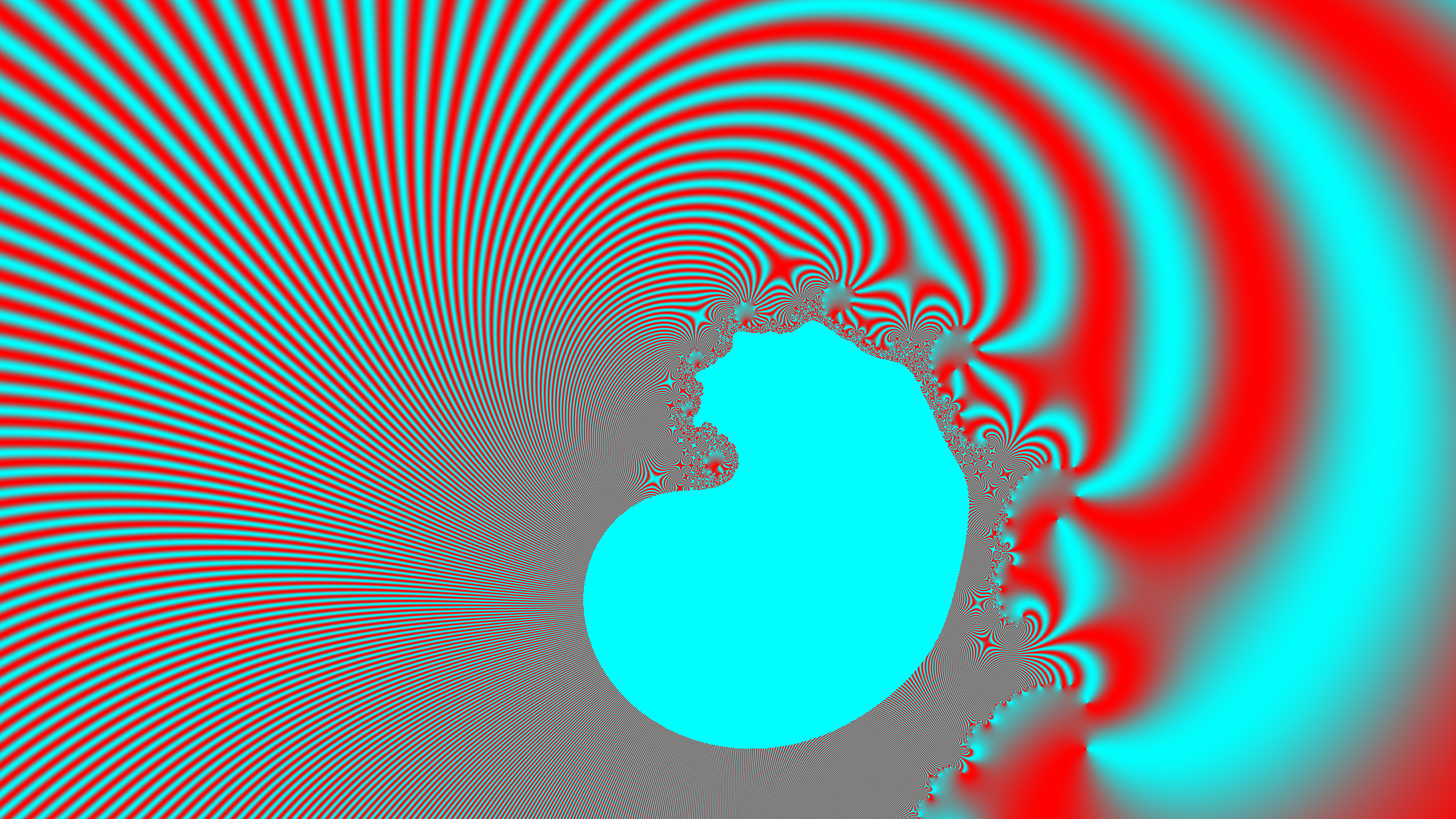
//
// fractal.c - written by Ted Burke - 8-Mar-2023
//
// gcc -o fractal fractal.c -lm
//
#include <stdio.h>
#include <complex.h>
#include <stdlib.h>
#include <stdint.h>
#include <math.h>
#define W 1920
#define H 1080
uint8_t p[H][W];
int main()
{
int x, y, n;
complex double z, c, zz;
uint8_t *p = malloc(W*H*3);
c = 0.625 - 0.4*I;
for (y=0 ; y<H ; ++y)
{
for (x=0 ; x<W ; ++x)
{
z = 0.02 * (x-W/2 + I*(y-H/2));
for (n=0 ; n<16 ; ++n)
{
zz = cpow(z, M_PI);
z = (z*z * c)/(z + c);
}
//uint8_t pp = 255 / (1.0 + cabs(z));
uint8_t pp = 127 * (1.0 + cos(carg(z)));
p[3*(y*W+x)+0] = 255 - pp;
p[3*(y*W+x)+1] = pp;
p[3*(y*W+x)+2] = pp;
}
}
FILE *f = fopen("fractal.pgm", "wb");
fprintf(f, "P6\n%d %d\n255\n", W, H);
fwrite(p, W*H*3, 1, f);
fclose(f);
free(p);
return 0;
}