_ _
(_) ___ | |_ ____
| |/ _ \| __|_ /
| | (_) | |_ / /
_/ |\___/ \__/___|
|__/
v a p o r w a v e program - work in progress
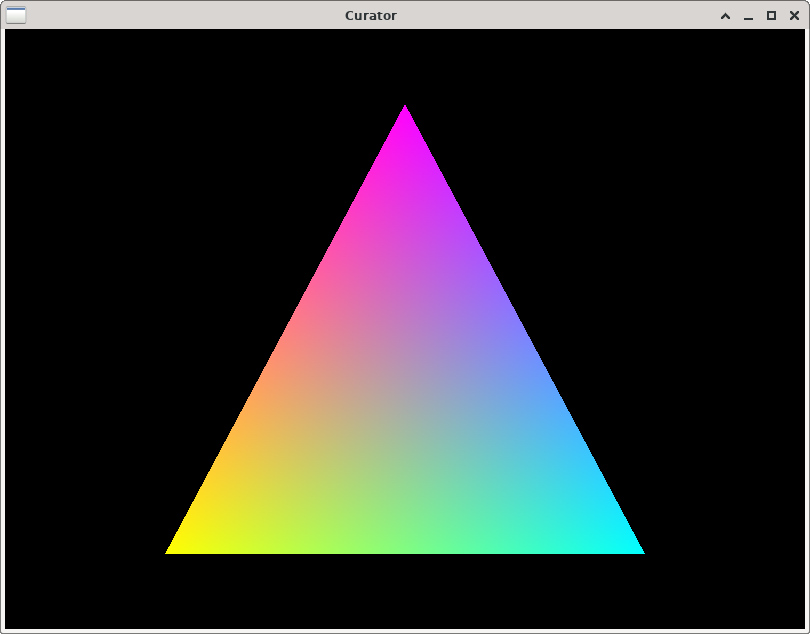
//
// v a p o r w a v e
// Written by Ted Burke 7-July-2022
//
// sudo apt install freeglut3-dev
// gcc main.c -lGL -lGLU -lglut
// (Note: -lGLU isn't actually required for initial example)
//
// Notes on compiling OpenGL / glut programs:
// https://web.eecs.umich.edu/~sugih/courses/eecs487/glut-howto/
//
// OpenGL examples: https://cs.lmu.edu/~ray/notes/openglexamples/
// Learn OpenGL shaders: https://learnopengl.com/Getting-started/Shaders
//
#include <stdio.h>
#include <GL/glut.h>
void display1()
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_POLYGON);
glColor3f(1, 0, 0); glVertex3f(-0.6, -0.75, 0.5);
glColor3f(0, 1, 0); glVertex3f(0.6, -0.75, 0);
glColor3f(0, 0, 1); glVertex3f(0, 0.75, 0);
glEnd();
glFlush();
}
void display2()
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_POLYGON);
glColor3f(1, 1, 0); glVertex3f(-0.6, -0.75, 0.5);
glColor3f(0, 1, 1); glVertex3f(0.6, -0.75, 0);
glColor3f(1, 0, 1); glVertex3f(0, 0.75, 0);
glEnd();
glFlush();
}
int main(int argc, char** argv)
{
GLint win1, win2;
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowPosition(80, 80);
glutInitWindowSize(400, 300);
win1 = glutCreateWindow("Player");
glutDisplayFunc(display1);
glutInitWindowPosition(160, 160);
glutInitWindowSize(800, 600);
win1 = glutCreateWindow("Curator");
glutDisplayFunc(display2);
glutMainLoop();
}